course
JavaScript Development
Learn to write professional software in JavaScript
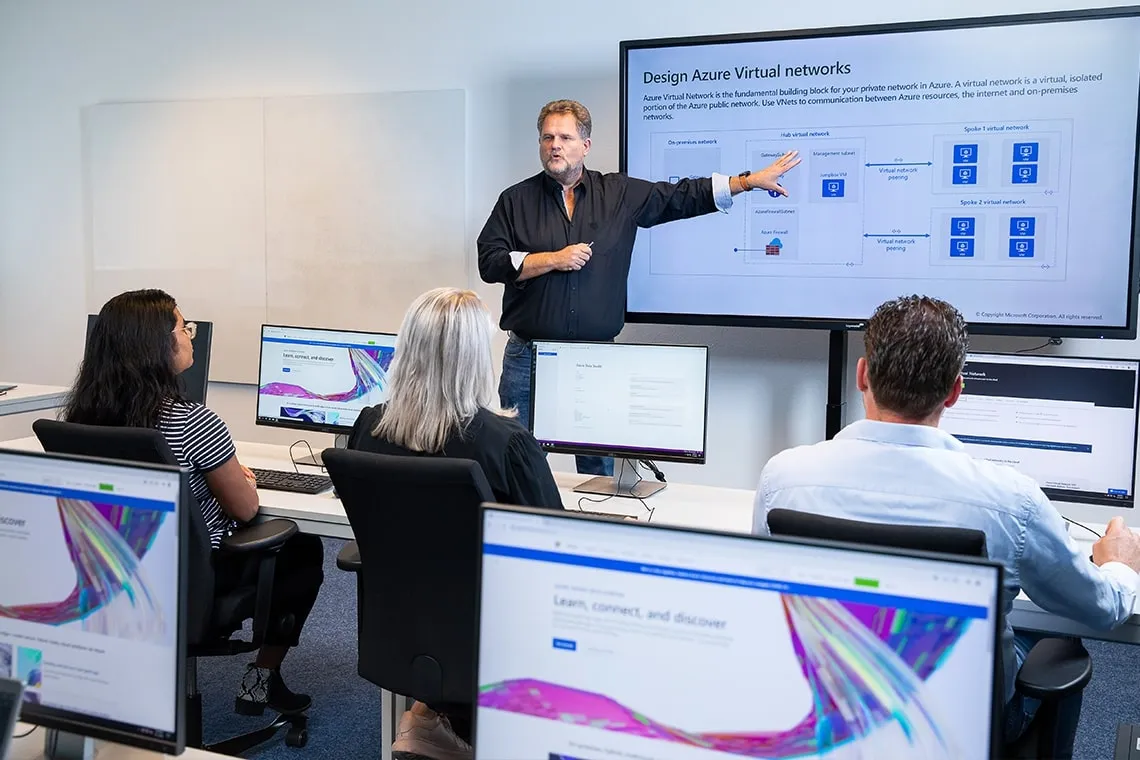
Description
JavaScript is currently one of the most popular languages to program in. What once began as "adding some interactivity to the browser" has grown into a language that's used for frontend with web applications and apps as well as backend.
And yet, JavaScript is an unknown, underappreciated, misunderstood and underestimated language. In its syntax, it much resembles Java, but it has its own very distinct semantics. JavaScript has a simple, dynamic and flexible type system. It doesn't have the classes, inheritance or encapsulation that we're used to in traditional OO-languages. Furthermore, JavaScript has influences from functional programming, strange scoping rules, and operators that differ treacherously from Java/C#. This all leads us to a very different world than the one we're traditionally used to with Java/C#/C++.
For a long time, it was sufficient to know only the basics of JavaScript in order to write small functions and handle events in a browser. But with the arrival of modern 'rich clients', that has changed. A solid and professional understanding of the JavaScript language in necessary to utilize its full power and to avoid its pitfalls.
You will become familiar with the type system of JavaScript and the role of its operators. You will find out which operators and control structures work different compared to C#/Java/C++. You will practice with lexical scoping and learn to use the similarities (and differences) between functions, arrays, and objects. You will also learn about often used techniques and constructions, like destructuring, classes and modules.
Advanced language features and techniques will also be covered. This knowledge and these skills will come very much in handy now that more and more projects are seeking to leverage the strength of JavaScript. It also aids on projects with React, Svelte, TypeScript or test frameworks like Cypress and Playwright where more advancted techniques come into play for structuring code. This is why we will go onto great detail about the advanced use of functions like arrow functions and closures, the advanced use of objects with encapsulation and proxies, in what ways JavaScript's inheritance mechanism can be an asset, when Symbols are useful, when Set
s and Map
s can work to your advantage, how iterators and generators offer interesting perspectives for loops and finally, everything about the wrapping of asynchronous work with Promises.
Did you know that, in JavaScript:
- it's true that:
"1" == true
++x
doesn't always result in the same value asx+1
- There are no public or private access modifiers, but there is a syntax for getters and setters
- Objects, functions and arrays look very much alike
- The reflection API is more or less built into the language
- Semicolon insertion is often useful, but not always
- Starting with ECMAScript 2015, a lot of existing design patterns have been included as language features. This is why we extensively cover the new versions of ECMAScript. Among other topics, you'll learn new syntaxes, patterns and possibilities. Examples of these are block bindings, destructuring, arrow functions, classes and modules
This course is meant for developers with a working knowledge of C#, Java, or C++, that will use JavaScript in a professional environment.
Prior Knowledge
Basic programming skills.
Subjects
Types
In this module, you will learn about JavaScript's type system.
Lessons
- Built-in types
- Primitive types like
number
,string
,null
,undefined
, etc. - Reference types and built-in objects such as
globalThis
- Regular expressions
- Variable declaration and their scope
After completing this module, students will be able to:
- Understand how JavaScript handles types
- Know the difference between
var
,let
enconst
Conversions
In this module, you will learn everything about JavaScript's conversions
Lessons
- When conversions take place
- JavaScript's preferences with conversions
Object
/Array
tostring
/number
number
tostring
string
tonumber
After completing this module, students will be able to:
- Understand when conversions take place
- Understand how objects and primitive values are converted
Operators
In this module, you will learn about JavaScript's operators
Lessons
==
vs===
typeof
,instanceof
,delete
, etcx ? y : z
,...
,||
,&&
,??
,**
, etc+
,**
,??=
,||=
, etc
Lab: types, conversions and operators
After completing this module, students will be able to:
- Name the difference between
&&
,||
and??
- Recognize all operators and know their usefulness
Control structures
In this module, you will learn about JavaScript's control structures
Lessons
- Standard control structures like
for
,if
andwhile
switch
for..in
andfor..of
- Exception handling
- Strict mode
- Semicolon insertion
After completing this module, students will be able to:
- Know when to use
in
andof
withfor
-loops - Throwing and handling exceptions
- Taking advantage of strict mode
- Determine whether you're pro-semicolons or against
Arrays
In this module, you will learn to work with arrays and that arrays in JavaScript secretly aren't arrays
Lessons
- Create and use arrays
- That JavaScript arrays are not your typical arrays
- Use Array functions
- Use the spead-operator with arrays
Lab: Arrays
After completing this module, students will be able to:
- Interact with arrays on a professional level
Objects
In this module, you will learn to create, use and otherwise interact with objects
Lessons
- Create objects
- Object literals
- Different notations for accessing properties
- Duplicate properties and its applications
- Using the
JSON
-object
Lab: Objects
After completing this module, students will be able to:
- Handle objects in a professional manner
- Create factories
- Convert objects to JSON and vice versa
Functions
In this module, you will learn to professionally interact with functions
Lessons
- The hoisting of functies
- Function parameters and default parameters
- Block-level functions
- Arrow functions:
() => {}
Lab: Functions
After completing this module, students will be able to:
- Professionally handling functions
- Use function parameters and default parameters
- Determine when to use "regular" functions and when to use an arrow function
Destructuring
In this module, you will learn how to destruct objects and arrays
Lessons
- Destructuring objects
- Destructuring arrays
- Destructuring an object/array-combination
- Set default values and aliases
- Using the rest-operator in combination with destructuring
- Practical applications of destructuring
Lab: Destructuring
After completing this module, students will be able to:
- Destructure a complex object or array
- Recognize when a situation practically lends itself for destructuring
Classes
In this module, you will learn to professionally handle classes
Lessons
- Define and use classes
- Class expressions
- Getters/setters
static
- Inheritance
- Overriding functions
- Abstract classes
Lab: Classes
After completing this module, students will be able to:
- Define and use classes
- Write getters/setters on a class
- Define
static
functions and properties - Apply inheritance
- Make a class functionally abstract
Modules
In this module, you will learn to work in a modular fashion using ES Modules
Lessons
- Why modules
- Modules in the browser
import
,export
and their variations- Getting acquainted with module bundlers
Lab: Modules
After completing this module, students will be able to:
- Work in a modular fashion using ES Modules
- See the advantages of module bundlers
Advanced functions
In this module, you will learn about the advanced use of functions
Lessons
- Function properties
- Constructor functions
- The mystery of
this
unveiled - Arrow functions
- IIFE's
- Closures
- Template literals
Lab: Advanced functions
After completing this module, students will be able to:
- Determine when to use a function and when to use an arrow function
- Reason about the value of
this
- Utilize the advantages of arrow functions
- Understand libraries better an appropriate situation presents itself
Prototypes
In this module, you will learn what prototypes are and how you can apply them
Lessons
- Prototypes
- Prototype chain
- Set/change the prototype
- Inheritance
super
Lab: Prototypes
After completing this module, students will be able to:
- Understand how prototypes work in JavaScript
- Use prototypes for inheritance of writing extension methods
- Understand how classes relate to prototypes8
Symbols
In this module, you will learn about Symbols: a value-type to uniquely address something
Lessons
- Symbols
- The value type
- Built-in symbols and the symbol registry
- Symbols and properties
- Libraries and symbols
Lab: Symbols
After completing this module, students will be able to:
- Use and write symbols
- Influence JavaScript's conversions using
Symbol.toPrimitive()
Sets and maps
In this module, you will learn about the built-in types Set
and Map
Lessons
Set
enMap
- Their weaker counterparts,
WeakSet
enWeakMap
Lab: Set
s en Map
s
After completing this module, students will be able to:
- Use
Set
andMap
when an appropriate situation presents itself
Iterators and generators
In this module, you will learn about iterating using iterators and generators
Lessons
- Iterators,
Symbol.iterator()
and the Iterator pattern - Generators and
yield
Lab: Iterators and generators
After completing this module, students will be able to:
- Use iterators en generators
- Implement the iterator pattern
- Create a generator
Advanced objects
In this module, you will learn using objects in an advanced manner
Lessons
- Static functions on objects:
Object.is()
,Object.assign()
,Object.keys()
and more Proxy
Reflect
- Encapsulation
- Garbage collection
Lab: Advanced objects
After completing this module, students will be able to:
- Use certain static functions when an appropriate situation presents itself
- Use
Proxy
to trap interactions - Use
Reflect
for reflection-like functions - Shield functions/properties in various ways from other bits of code
- Hook into the event of an object being garbage collected by the JavaScript-engine
Promises
In this module, you will learn about performing asynchronous work using promises
Lessons
- Creating and using promises
- Promise chaining
- Error handling
- Returning values
- Static functions like
Promise.all()
,Promise.allSettled()
and more - Useful techniques
async
/await
Lab: Asynchronously read the contents of multiple files
After completing this module, students will be able to:
- Create and use promises
- Implement error handling and chaining
- Deal with parallel promises
- Use
async
/await
to improve readability
Schedule
Start date | Duration | Location | |
---|---|---|---|
April 28, 2025April 29, 2025April 30, 2025May 1, 2025May 2, 2025 | 5 days | Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information | Sign up |
July 28, 2025July 29, 2025July 30, 2025July 31, 2025August 1, 2025 | 5 days | Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information | Sign up |
All courses can also be conducted within your organization as customized or incompany training.
Our training advisors are happy to help you provide personal advice or find Incompany training within your organization.
Trainers
Prior knowledge courses
Follow-up courses
Introductie in de BlockChain technologie
Learn how to use BlockChain technology and understand the concepts.
- General
Node.js
Serverside JavaScript done right
- Web Development
Clean Code: Mastering the Art of Software Craftsmanship
Improve your programming skills and write more maintainable code
- C#/.NET
- Java/JVM
End-to-end testing with Cypress
Using the browser to test that your web application does what it should do
- Testing
Develop Applications Using the ReactJS Framework
Develop powerful and maintainable web applications using the latest techniques and best practices in ReactJS.
- Web Development
Angular: Building professional Single Page Applications
Are you a developer who wants to learn how to build a real-world Angular application, including TypeScript and testing? Then, this is the training for you.
- Web Development
Web Components
Use Web Components to build a web application
- Web Development
End-to-end testing with Playwright
Using the browser to test that your web application does what it should do
- Testing
Pragmatic JavaScript
Applying JavaScript in practice
- Web Development
TypeScript Development
Learn to develop maintainable JavaScript applications with TypeScript
- Web Development
Building a SPA with .NET Core, Vue and Identity Server
Learn how to build a Single Page Application using .NET Core Web API, Vue and Identity Server in a practical manner
- C#/.NET
- Web Development
"Very pleasant teacher, gave a very good interpretation of the course in their own way. It was nice to follow the course like that."Marieke
-
Hoge waardering
-
Praktijkgerichte trainingen
-
Gecertificeerde trainers
-
Eigen docenten