course
Programming with C#
Learn how to develop C# applications for the .NET platform
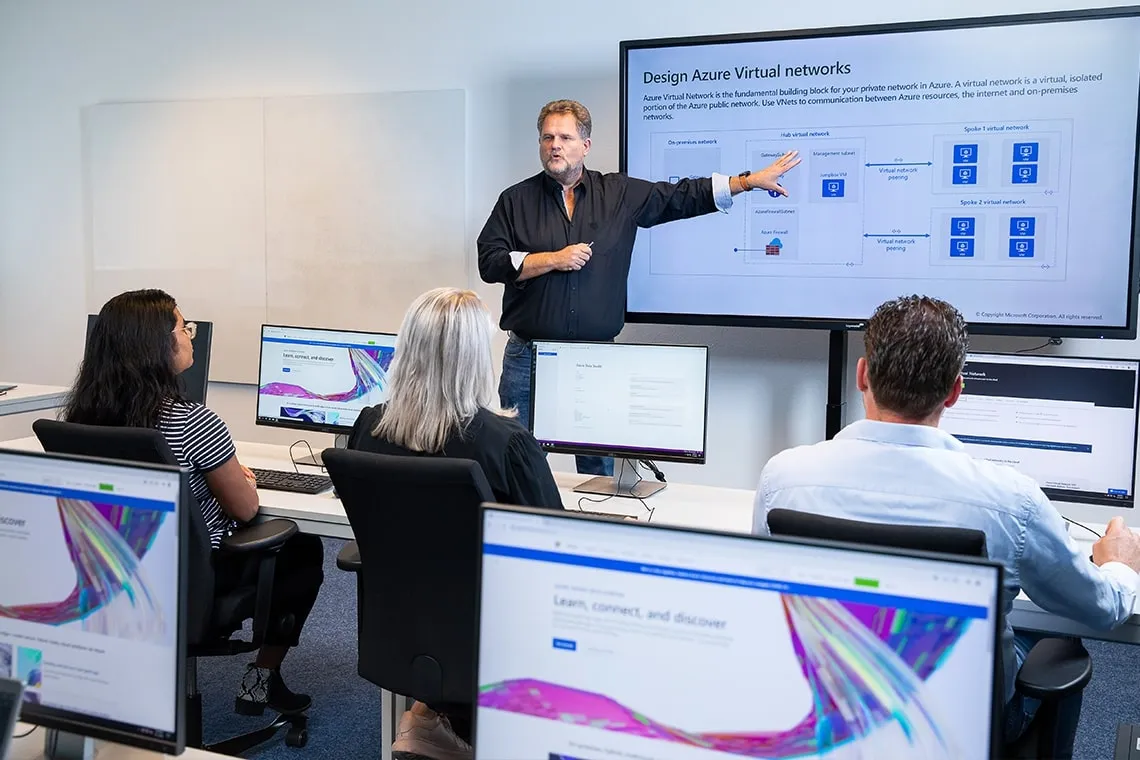
Description
If you want to build applications in .NET, it's essential to learn the most important .NET language: C#.
In this training you learn almost every keyword en language concept up to C# 10 and .NET 6.
You get not only the theory but also many chances to practice it for yourself. The exercises and the demos use Visual Studio, so that you can also learn how to use this powerful tool.
If you are a professional developer that already has experience in other languages and you want to get to know C#, this fully packed course is for you.
Learning Goals
Prior Knowledge
Experience with a 3GL programming language. Some familiarity with Object Orientation would help.
Subjects
Overview of the Microsoft .NET Platform
- Introduction to the .NET Platform
- .NET Implementations
- Languages in the .NET Framework
- History of .NET
- .NET (Core) vs .NET Framework
Overview of C#
- Getting Started
- From Source Code to IL
- From IL to Native
- Hello World
- Top Level Statements (C# 9)
- Basic Input/Output Operations
- Commenting
- Running, and Debugging
Type System
- Simple Types
- Tuple
- Built-in Reference Types
- Operators
- Creating User Defined Types
- Conversion
Statements and Exceptions
- Introduction to Statements
- Statements Blocks
- Types of Statements
- Selection Statements
- Iteration Statements
- Jump Statements
- Exceptions
Methods and Parameters
- Methods
- Local Variables
- Parameters
- Returned Values
- Overloaded Methods
- Expression Bodied Methods
- Local Functions
Arrays
- Overview of Arrays
- Creating Arrays
- Using Arrays
Object-Oriented Programming
- Principles of Object Orientation
- Classes
- Objects
- The
this
keyword
Using Reference-Types
- Difference between Value Types and Reference Types
- Declaring And Releasing
- Invalid References
- Comparing
- Passing
- The
using
syntax - Boxing and Unboxing
Creating and Destroying Objects
- Using Constructors
- Initializing Data
- Fields
- Properties
- Objects and Memory
- Resource Management
Properties, Indexers and Initializers
- Properties
- Indexers
- Initializers
Inheritance in C#
- Principles of Object Orientation
- Inheritance
- Virtual
- Override
- Abstract
- Sealed
- Interfaces
- Implementation
- Conversion
Namespaces, Assemblies and Visibility
- Visibility and Modifiers
- Aggregation
- Factories
- Namespaces
- Assemblies
Delegates and Events
- Delegates
- Anonymous delegates
- Lambdas
- Events
Generics
- Generic classes and methods
- constraints
If time permits:
Attributes and Conditional Compilation
- Attributes
- Conditional Compilation
Anonymous Types and Partial Methods
- Anonymous Types
- Partial Classes
- Partial Methods
Records
- Records
- Value Based Equality
- Support for Printed Output
- With-Expressions
- Inheritance
- Positional Records
Pattern Matching
- Pattern Matching
- Type Pattern
- Property Pattern
- Positional Pattern
- Recursive Pattern
- Tuple Pattern
Schedule
Start date | Duration | Location | |
---|---|---|---|
July 14, 2025July 15, 2025July 16, 2025July 17, 2025July 18, 2025 | 5 days | Veenendaal / Remote This is a hybrid training and can be followed remotely. More information Veenendaal / Remote This is a hybrid training and can be followed remotely. More information Veenendaal / Remote This is a hybrid training and can be followed remotely. More information Veenendaal / Remote This is a hybrid training and can be followed remotely. More information Veenendaal / Remote This is a hybrid training and can be followed remotely. More information | Sign up |
August 25, 2025August 26, 2025August 27, 2025August 28, 2025August 29, 2025 | 5 days | Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information Utrecht / Remote This is a hybrid training and can be followed remotely. More information | Sign up |
All courses can also be conducted within your organization as customized or incompany training.
Our training advisors are happy to help you provide personal advice or find Incompany training within your organization.
Trainers
Prior knowledge courses
Follow-up courses
Unit Testing with C#
Be more productive by practicing Test-Driven Development (TDD), leveraging advanced tools and frameworks, and applying specialized code patterns.
- C#/.NET
- Testing
C# Hands-on
During this case, you will use C# to solve various problems, starting with some simple string manipulation and finally performing complex tree manipulation.
- C#/.NET
Design Patterns for C++, C#, VB.NET and Java Developers
Learn to apply Design Patterns
- General
LINQ: .NET Language-Integrated Query
Learn LINQ, lambda expressions, extension methods and comprehension syntax.
- C#/.NET
Developing with Reqnroll
Increase your practical knowledge of Specification by Example with Reqnroll (the successor to SpecFlow)
- Requirements
- C#/.NET
- Testing
Developing Microsoft Blazor Web Applications
Learn how to build a Web Application using Microsoft Blazor in a practical manner
- C#/.NET
- Web Development
Clean Code: Mastering the Art of Software Craftsmanship
Improve your programming skills and write more maintainable code
- C#/.NET
- Java/JVM
Multithreading, Parallel Programming and Asynchronous Programming in C# .NET
Learn about Threads, Task Parallel Library and async / await
- C#/.NET
Developing ASP.NET Core Web Applications using Razor Pages and Blazor Components
Learn to develop advanced Microsoft ASP.NET Core Razor Pages applications with Blazor Components.
- C#/.NET
- Web Development
Building a SPA with .NET Core, Vue and Identity Server
Learn how to build a Single Page Application using .NET Core Web API, Vue and Identity Server in a practical manner
- C#/.NET
- Web Development
JavaScript Development Core
Learn professional JavaScript development
JavaScript Development
Learn to write professional software in JavaScript
- Web Development
"Very pleasant teacher, gave a very good interpretation of the course in their own way. It was nice to follow the course like that."Marieke
-
Hoge waardering
-
Praktijkgerichte trainingen
-
Gecertificeerde trainers
-
Eigen docenten