training
Unit Testing in Python
Wees productiever door Test-Driven Development (TDD), door advanced tools en frameworks te gebruiken en best practices voor unit testing toe te passen
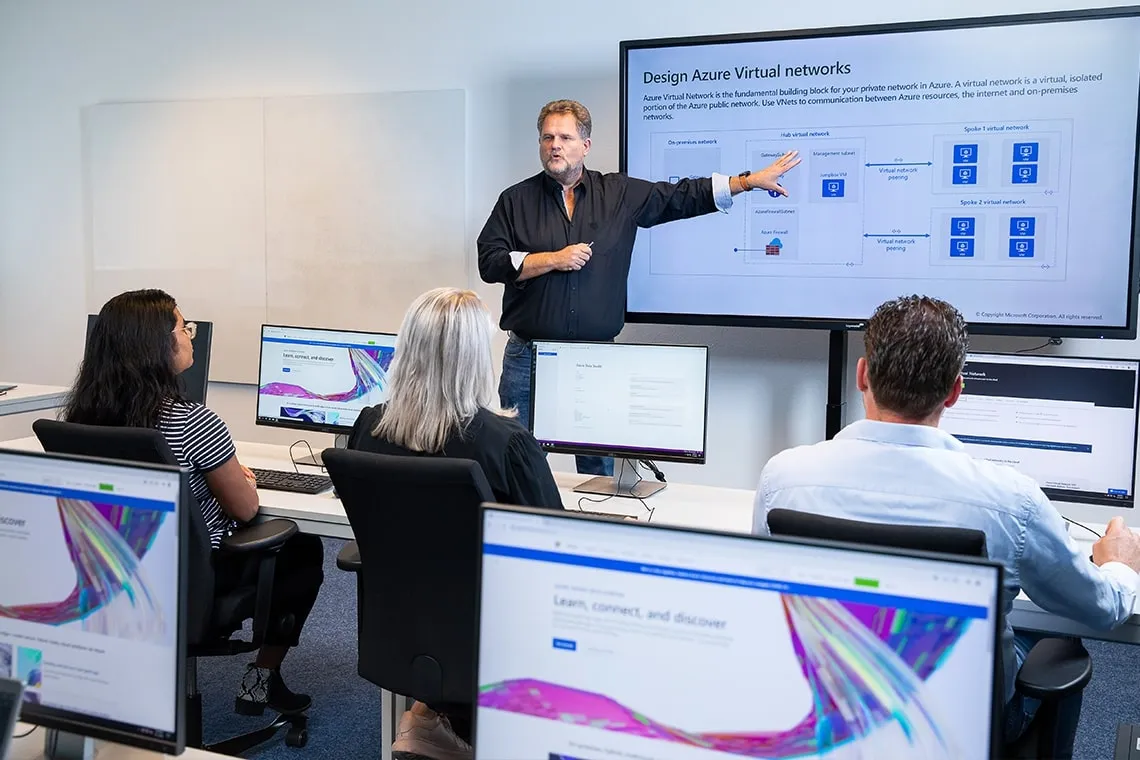
Beschrijving
In deze praktische training leren we je alles wat je moet weten om effectieve en onderhoudbare unit tests te schrijven met Python.
Je leert hoe je Test Driven Development (TDD) kunt gebruiken om bugs vroegtijdig te ontdekken, evenals wanneer je black-box versus white-box testing moet gebruiken. We duiken ook in de wereld van mocking met de unittest.mocking library, waarbij je leert waarom en hoe je dit effectief kunt gebruiken. Je leert over metrics zoals code coverage en mutation testing, en we zullen best practices bekijken die je helpen om efficiënte en hoogwaardige tests te schrijven.
We sluiten af door implementatie van integratietests met Testcontainers, zodat je er zeker van kunt zijn dat jouw code naadloos samenwerkt met andere onderdelen van je applicatie.
Na het volgen van deze training ben je goed uitgerust om unit tests te schrijven die bugs vroegtijdig opsporen, makkelijker te onderhouden zijn en je een betere Python-ontwikkelaar maken.
Leerdoelen
Benodigde voorkennis
Kennis van programmeren in Python
Onderwerpen
- About testing
- Unit testing basics
- Test Driven Development (TDD)
- Concept of mocking
- Code coverage
- Mocking with unittest.mock
- Testing frameworks
- Best practices
- Mutation testing
- Integration testing
About testing
In this chapter, you will learn the basics of testing. Why we test and what kind of tests there are.
Goals:
- Know the difference between linting and testing.
- Learn how to categorize all the different kinds of tests.
- Know the testing pyramid and how it can help you to reason about test levels.
- Learn the common anti-patterns and how to recognize them
Unit testing basics
In this chapter, we'll learn the basics of unit testing using the practical example.
Goals:
- Learn how to define the 'unit' to test.
- Learn the difference between white-box and black-box testing.
- Learn to recognize and use naming strategies for our tests.
- Know how to determine when you are 'done' with unit testing.
- Learn basic best practices
- Lab: implement unit tests for a simple library.
Test Driven Development (TDD)
In this chapter, you will learn what TDD is by example.
Goals:
- Learn TDD using the red-green-refactor cycle.
- Lab: Implement a simple library function in a TDD way
Concept of mocking
In this chapter, you will learn what 'mocking' entails and identify where you should use it by identifying your 'system under test' (SUT) and splitting from your 'dependencies'
Goals:
- Learn to identify the SUT from its dependencies
- Learn how to write a mock by hand
- Understand the need for dependency injection when working with mocks.
- Lab: implement dependency injection and setup mocking using classes.
Code Coverage
In this chapter, we will focus on testing metrics, namely code coverage.
Goals:
- Know what the difference is between code coverage and mutation testing.
- Know what kinds of coverage metrics there are and how to choose between them.
- Learn the benefits of a quick feedback cycle with code coverage.
- Learn how to run code coverage on our code using the command line.
- Know how to 'break the build' when coverage is too low.
- Lab: use code coverage to improve our tests.
Mocking with unittest.mock
In this chapter, you will learn to use a mocking library. We will focus on unittest as an example.
Goals:
- Learn what monkeypatching is and how to use it.
- Learn the API of unittest.mock; how to create mocks, configure them using
.side_effect
and verify behavior using.assert()
- Lab: Implement your tests using unittest.mock
Testing frameworks
In this chapter, we will focus on the most popular testing framework in python: PyTest
Goals:
- Know what the benefit is of PyTest
- Learn how it extends unittest
- Learn to parameterize our tests.
- Know what common pitfalls are and how to avoid them.
- Lab: Improve our test maintainability using features of the testing framework.
Best practices
In this chapter, we will look at some best practices we can use to improve the maintainability of our tests further.
Goals:
- Know how to identify tests that are too DRY (Don't Repeat Yourself) or too WET (We Like Typing).
- Learn to prefer DAMP tests (Descriptive and Meaningful Phrases).
- Know when to use a builder pattern
- Know where to find more best practices
- Lab: Implement DAMP tests using the builder pattern.
Mutation testing
In this chapter, we will focus on testing metrics, namely mutation testing.
Goals:
- Know what the difference is between code coverage and mutation testing
- Understand how mutation testing works conceptually.
- Learn what the "mutation score" metric is
- Learn how to use mutmut to run mutation testing on python code.
- Know how to read a mutation testing report.
- Know how to 'break the build' when the mutation score is too low.
- Lab: Improve the effectiveness of your tests using mutmut.
Integration testing
In this chapter, we'll be 'scaling up the pyramid': Welcome to integration testing.
Goals:
- Be able to identify where an integration test is needed.
- Learn to identify 'shared state' in dependencies.
- Learn how to use TestContainers to create dependencies on the fly.
- Know what the benefits are of using TestContainers
- Learn to organize integration tests inside your project structure.
- Lab: Implement integration tests for a database using TestContainers
Planning
Startdatum | Duur | Locatie | |
---|---|---|---|
10 juni 202511 juni 2025 | 2 dagen | Veenendaal / Remote Dit is een hybride training die remote gevolgd kan worden. Meer informatie Veenendaal / Remote Dit is een hybride training die remote gevolgd kan worden. Meer informatie | Inschrijven |
24 juli 202525 juli 2025 | 2 dagen | Veenendaal / Remote Dit is een hybride training die remote gevolgd kan worden. Meer informatie Veenendaal / Remote Dit is een hybride training die remote gevolgd kan worden. Meer informatie | Inschrijven |
6 oktober 20257 oktober 2025 | 2 dagen | Utrecht / Remote Dit is een hybride training die remote gevolgd kan worden. Meer informatie Utrecht / Remote Dit is een hybride training die remote gevolgd kan worden. Meer informatie | Inschrijven |
Incompany of persoonlijk advies nodig?
Onze opleidingsadviseurs denken graag met je mee om een persoonlijk advies te geven of een incompany training binnen jouw organisatie te vinden.
Trainers
Voorkennis trainingen
"Trainer die zijn vak kent!"Marc
-
Hoge waardering
-
Praktijkgerichte trainingen
-
Gecertificeerde trainers
-
Eigen docenten